A very common problem people encounter when working with IE in VBA is VBA attempting to run code before Internet Explorer has fully loaded. By using this code, you tell VBA to repeat a loop until IE is ready (IE.ReadyState – 4). The Rich Textbox control does not come with MS Office - it is a VB6 control, so it may not be present on all PCs. Moreover, Microsoft has disabled its use in Office applications because of security problems - see Problems occur when you use the Rich TextBox Control 6.0 in Office XP and in Office 2003. The suggested workaround is to use VB6 to.
Excel Vba Microsoft Internet Controls Reference
I have been working VBA to scrape website HTML code using the Microsoft HTML Object Library and the Microsoft Internet Controls Library. Now that support for Internet Explorer is being phased out, I am trying to switch my code over to scrape Google Chrome using the Selenium Type Library which is an open source download. Step 1: In the VBA editor (ALT + F11), create a userform as usual: Right click at any of the objects and then insert - UserForm. Step 2: In order to add the Webbrowser object you should do the following: Right click on the toolbox and select Additional Controls. Next, from the available choices, select Microsoft Web Browser and then click OK.
I've shamelessly stolen my colleague Andrew's idea to create this blog, showing how to fill in an Internet Explorer (IE) form from within VBA. I've also shamelessly borrowed ideas from Fergus Cairns - thanks, Fergus.
We'll create a macro in VBA to fill in the search form on the old Wise Owl site (now superseded, but the principle remains the same).
Note that our website has changed since this blog was written, so while the principle of the code shown below is still good, it won't work on our site. I've published a newer blog on the same subject here - or you can see a full list of all of our VBA training resources here.
Understanding the Target Page
The first thing to do is to know what the elements on the target website page are called. To do this, load the website in IE and view its source HTML:
In Internet Explorer 9, this is how you view source HTML using the right mouse button menu.
If you press CTRL + F to find some known text (eg Search for this page), you should be able - eventually - to track down the part of the form of interest:
The HTML for the search button on the Wise Owl website.
See the end of this blog for the BBC and Google UK search form field names.
From the above we can see that the search form with id search contains two controls which a user can interact with:
- A textbox called SearchBox; and
- A button called submit2.
Now that we know what we're trying to look to in the Document Object Model, we can start writing our VBA.
Referencing the Microsoft Internet Controls
The next thing to do is to make sure that we can get VBA to talk to Internet Explorer. To do this, reference the object library called Microsoft Internet Controls (if you're not sure what references are, read this first).
First, in VBA create a reference:
Choose the option shown from the VBA code editor (this example is for Excel).
Select Microsoft Internet Controls (you may find it helpful to type an M in first, to go down to the object libraries starting with M):
Tick the Microsoft Internet Controls object library reference
Select OK. If you choose the menu option again, you'll see this reference has moved to near the top of the list:
The libraries referenced always include Excel. The one you just chose appears near the top of the list.
The Code to Link to IE
You can now write the code to link to Internet Explorer - here's a suggestion:
Sub UseInternetExplorer()
'Make sure you've set a reference to the
'Microsoft Internet Controls object library first
'create a variable to refer to an IE application, and
'start up a new copy of IE (you could use GetObject
Excel Vba Microsoft Internet Controls Examples
'to access an existing copy of you already had one open)
Dim ieApp AsNew SHDocVw.InternetExplorer
'make sure you can see this new copy of IE!
ieApp.Visible = True
'go to the website of interest
ieApp.Navigate 'Wise Owl or other website address goes here'
'wait for page to finish loading
DoWhile ieApp.busy
Loop
'get a reference to the search form, by finding its id in the
'web page's document object model
Dim ieElement AsObject
Set ieElement = ieApp.document.getElementByID('search')
'search box is composed of text box (item 0) and button (item 1)
'set value of text box to what we're searching for
ieElement(0).Value = 'Excel VBA courses'
'click the button!
ieElement(1).Click
EndSub
If you run this macro, it should search the Wise Owl site for information on Excel VBA courses!
Except that it might not - timing is everything. You may need to delay your code to wait for Internet Explorer or your website to catch up - see below.
Delaying your code
Unfortunately, code runs really quickly and websites load slowly (at least relatively), so you often need to slow your code down with loops like this:
'wait for page to finish loading
DoWhile ieApp.Busy
DoEvents
Loop
Or possibly even this:
'wait for page to finish loading
DoWhile ieApp.Busy And Not ieApp.ReadyState = READYSTATE_COMPLETE
DoEvents
Loop
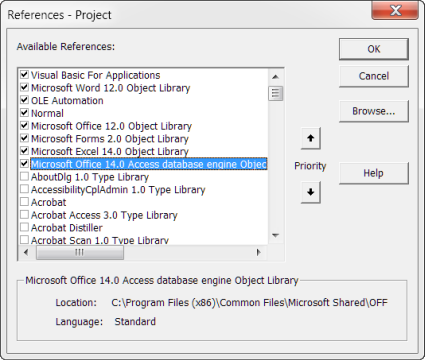
It seems to be more or less guesswork how many you put in!
BBC and Google Help
Fergus Cairns informs me that you can substitute the following terms for the BBC website and Google UK:
Website | Search box | Find button |
---|---|---|
Google UK | gbqfq | btnG |
BBC UK | orb-search-q | orb-search-button |
So at the time of writing this (September 2014) the following code works - usually!
Sub FillInBBCSearchForm()
'Make sure you've set a reference to the
'Microsoft Internet Controls object library first
'create a variable to refer to an IE application
Dim ieApp AsNew SHDocVw.InternetExplorer
'make sure you can see this new copy of IE!
ieApp.Visible = True
'go to the website of interest
ieApp.Navigate 'http://www.google.co.uk'
DoWhile ieApp.Busy
DoEvents
Loop
'wait for page to finish loading
DoWhile ieApp.Busy And Not ieApp.ReadyState = READYSTATE_COMPLETE
DoEvents
Loop
'fill in the search form
ieApp.Document.getElementById('gbqfq').Value = 'Excel VBA'
'wait for page to finish loading
DoWhile ieApp.Busy
DoEvents
Loop
'wait for page to finish loading
DoWhile ieApp.Busy And Not ieApp.ReadyState = READYSTATE_COMPLETE
DoEvents
Excel Vba Microsoft Internet Controls Diagram
Loop
'click on the search button
ieApp.Document.all('btnG').Click
EndSub
Excel Vba Microsoft Internet Controls Free
Notice that I've put in a fairly random number of loops to slow this down!