The sample source code outlines the basics of communicating directly with an ADU device on Linux and OS X using Python and libhidapi. Basics of opening a USB device handle, writing and reading data, as well as closing the handle of the ADU usb device is provided as an example. Python usb.USBError Examples The following are 18 code examples for showing how to use usb.USBError. These examples are extracted from open source projects. You can vote up the ones you like or vote down the ones you don't like, and go to the original project or source file by following the links above each example.
In this python tutorial, we will discuss the Python copy file. We will also check:
- Python copy file to a folder
- Python copy files from one location to another
- Python copy file from one directory to another
- Python copy file to USB
- Python copy file content to another file
- Python copy file and rename
- Python copy file from subfolders to one folder
- Python copy file to the current directory
- Python copy file line by line
- Python copy file overwrite
Let us check on how to copy files in Python with a few examples.
Python shutil.copy()method
The shutil.copy() method in Python is used to copy the files or directories from the source to the destination. The source must represent the file, and the destination may be a file or directory.
This function provides collection and operations on the files it also helps in the copying and removal of files and directories.
Check out File does not exist Python.
Python copy file to folder
Now, we can see how to copy file in python.
- In this example, I have imported two modules called shutil, and os this module helps in automating the process of copying and removing files and directories.
- We have to write a path for both source and destination. We have to use shutil.copyfile(src, dst) to copy the file from source to destination. Along with the path, the name of the file with its extension should be written.
- src = r’C:UsersAdministrator.SHAREPOINTSKYDesktopWorkname.txt’ is the source path. name.txt is the filename that I have created with the .txt extension.
- dst = r’C:UsersAdministrator.SHAREPOINTSKYDesktopNewfoldername.txt’ is the destination path.Here, I have created a folder called Newfolder to copy the file name.txt in the Newfolder.
Example:
Python copy files from one location to another
Here, we can see how to copy files from one location to another in Python
- In this example, I have imported two modules called shutil and os. Here, the shutil module helps in automating the process of coping and removing files and directories.
- src= r’C:Newfilefile2.txt’ is the source path. file2.txt is the filename that I have created with the .txt extension.
- dst = r’D:file2.txt’ destination path. file2.txt is the filename that I have created with the .txt extension.
- Here, I have copied the file from C drive to D drive
- To copy the file from one location to another, we have to use shutil.copyfile(src, dst).
Example:
Python copy file from one directory to another
Here, we can see how to copy file from one directory to another in Python.
- In this example, I have imported modules called shutil and os in Python. The shutil module helps in automating the process of coping and removing files and directories.
- src = r’C:UsersAdministrator.SHAREPOINTSKYDesktopWorkfile2.txt’ is the source path. file2.txt is the name of the file with the .txt extension.
- dst = r’C:UsersAdministrator.SHAREPOINTSKYDesktopNewfolderfile2.txt’ is the destination path. file2.txt is the name of the file with the .txt extension.
- Work and New folder are the two directories.
- To copy the file from one directory to another, we have to use shutil.copyfile(src, dst).

Python Usb Example Code
Example:
Example:
Python copy file to USB
Here, we can see how to copy a file to USB in Python.
- In this example, I have imported modules called shutil and os. The shutil module helps in automating the process of coping and removing files and directories.
- I have used shutil.copy(src, dst) to copy the file to USB.
- src=r’C:UsersAdministrator.SHAREPOINTSKYDesktopWorkname.txt is the path of the file, name.txt is the filename that I have created with the .txt extension.
- dst=r’D:lakshminame.txt’ is the path of the USB. The name.txt is the filename that I have created with the .txt extension. In this “lakshmi” is the folder name and the filename is name.txt which is copied to “r’D:lakshminame.txt’ “ (which is our USB drive).
- Along with the path, the name of the file and its extension should be written.
Example:
This is how to copy file to USB in Python.
Python copy file content to another file
Now, we can see how to copy file content to another file in Python.
- In this example, I have imported two modules called os and sys. The sys module provides functions and variables used to manipulate the program.
- f = open(r’C:UsersAdministrator.SHAREPOINTSKYDesktopWorkemployee.txt’,”r”) is the source path. The employee.txt is the file name that I have created with the .txt extension.
- copy = open(r’C:UsersAdministrator.SHAREPOINTSKYDesktopNewfolderemployee.txt’,”wt”) is destination path. The employee.txt is the file name that I have created with the .txt extension.
- line = f.read() reads the specified bytes from the file to another file.
- copy.write(str(line)) is used to write each line in the file.
- f.close is used to close the opened file. It is necessary to use a copy.close() while iterating a file in python.
Example:
This is how we can copy file content to another file in Python.
Python copy file and rename
Here, we can see how to copy file and rename in Python.
- In this example, I have imported Python modules called shutil and os.
- Firstly, we have to copy the file from source to destination and then rename the copied file.
- src = r’C:UsersAdministrator.SHAREPOINTSKYDesktopWorkname.txt’ is the source path. name.txt is the file name that I have created with the .txt extension.
- dst = r’C:UsersAdministrator.SHAREPOINTSKYDesktopNewfoldername.txt’ is the destination path. The name.txt is the file name that I have created with the .txt extension.
- os.rename is used to rename the folder name. To rename the file, I have used os.rename(r’C:UsersAdministrator.SHAREPOINTSKYDesktopWorkname.txt’,r’C:UsersAdministrator.SHAREPOINTSKYDesktopNewfolderdetails.txt’)
- shutil.copyfile(src, dst) is used to copy the file from source to destination.
- The name.txt file name is now renamed by the name called details.txt.
Example:
The above code, we can use to copy file and rename in Python.
Python copy file from subfolders to one folder
Here, we will see how to copy file from subfolder to one folder in Python.
- In this example, I have used two modules called shutil, and os. The shutil module helps in automating the process of coping and removing files and directories.
- The os module includes many functionalities like interacting with the filesystem.
- src= r’C:UsersAdministrator.SHAREPOINTSKYDesktopWorkofficeemployee.txt’. is the source path, employee.txt is the filename that I have created with the .txt extension.
- dst = r’C:UsersAdministrator.SHAREPOINTSKYDesktopNewfolderemployee.txt‘. is the destination path. The employee.txt is the filename that I have created with the .txt extension.
- The office is a subfolder and a New folder is another folder in this example.
Example:
This is how to copy file from subfolders to one folder in Python.
Python copy file to the current directory
Now, we can see how to copy file to the current directory in Python.
Python Usb Camera Example
- In this example, I have used two modules called shutil, and os. The shutil module helps in automating the process of coping and removing files and directories.
- The os module includes many functionalities like interacting with the filesystem.
- src = r’C:UsersAdministrator.SHAREPOINTSKYDesktopWorkofficeemployee.txt’ is the source path. employee.txt is the filename that I have created with the .txt extension.
- dst = r’C:UsersAdministrator.SHAREPOINTSKYDesktopWorkemployee.txt’ is the destination path. The employee.txt is the filename that I have created with the .txt extension.
- Work is the current directory in this example.
- shutil.copyfile(src, dst) is used to copy the file to the current directory.
Example:
This is how to copy file to the current directory in Python.
Python copy file line by line
Now, we can see how to copy file line by line in python.
- In this example, I have imported two modules called os and sys. The sys module provides functions and variables used to manipulate the program.
- src = open(r’C:UsersAdministrator.SHAREPOINTSKYDesktopWorkemployee.txt’,”r”) is the source path. The employee.txt is the filename that I have created with the .txt extension.
- dst = open(r’C:UsersAdministrator.SHAREPOINTSKYDesktopNewfolderemployee.txt’,”wt”) is the destination path. The employee.txt is the filename that I have created with the .txt extension.
- I have used the iterating function to iterate the file object in the range i = 1 and i < 3. So, only 2 lines from the file will be copied.The copy.write(str(line)) is used to write a single string into the file.
- “r” mode is used to read the file, “wt” is used to write the file. The f.close is used to close the file.
- f.read returns the specified number of bytes from the file.
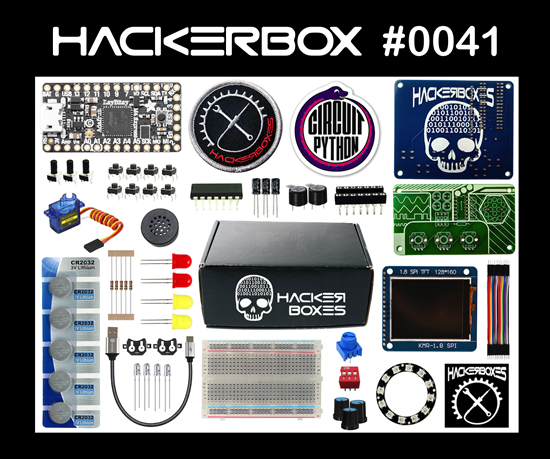
Example:
This is how to copy file line by line in Python.
Python copy file overwrite
Here, we can see how to copyfile and overwrite in python.
- In this example, I have imported a module called shutil, and os. The shutil module helps in automating the process of coping and removing files and directories.
- src = r’C:UsersAdministrator.SHAREPOINTSKYDesktopWorkemployee.txt’. is the path of the source file. The employee.txt is the filename that I have created with the .txt extension.
- dst = r’C:UsersAdministrator.SHAREPOINTSKYDesktopWorkemployee.txt’. is the path of the destination file. The employee.txt is the filename that I have created with the .txt extension.
- shutil.move(src, dst) is used to copy the file from source to destination.
- If the file is already present it will be moved to another directory, and then the file is overwritten. The shutil.move(src, dst) is used to move the file and overwrite. The work is the folder name.
Example:
Python check if a file is open
Here, we will see how to check the file is present or not by using os.system in python.
- In this example, I have imported a module called os.path. This module is a path module for the operating system. It is used for the local paths.
- The if os.path.isfile() is a method used to check whether the given files are present or not. The ‘kavita.txt’ is the filename. The else condition is used here to check if the condition is true, then it returns the file which is present else it will return the file not present.
Example:
As the file is present, It returns a file is present in the output. You can refer to the below screenshot for the output.
This is how to check if a file is open in Python.
Python check if a file is already opened
Here, we can see how to check if a file is already opened in python
In this example, I have opened a file called document using “wb+” mode which is used to readandwrite the file, as the file is created and it is already present it gives the output as the file is already opened. The ‘document’ is the file name.
Example:
To check the file is already opened, I have used print(“file is already opened”). You can refer to the below screenshot for the output.
This is how to check if a file is already opened in Python.
Python check if a file exists
Now, we can see how to check if a file exists in python.
- In this example, I have imported a module called os.path. This module is a path module for the operating system, it is used for the local paths.
- The path.exists() is a method that returns true if the file exists otherwise it returns false. Here, ‘document’ is the file name.
Example:
To check whether the file exists or not, I have used print(path.exists(‘document’)). The below screenshot shows the output.
The above coder, we can use to check if a file exists in Python.
You may like the following Python tutorials:
In this Python tutorial, we have learned about the Copy file in Python. Also, We covered these below topics:

- Python copy file to a folder
- Python copy files from one location to another
- Python copy file from one directory to another
- Python copy file to USB
- Python copy file content to another file
- Python copy file and rename
- Python copy file from subfolders to one folder
- Python copy file to the current directory
- Python copy file line by line
- Python copy file overwrite
Entrepreneur, Founder, Author, Blogger, Trainer, and more. Check out my profile.